Mastering Astro Components: A Comprehensive Tutorial
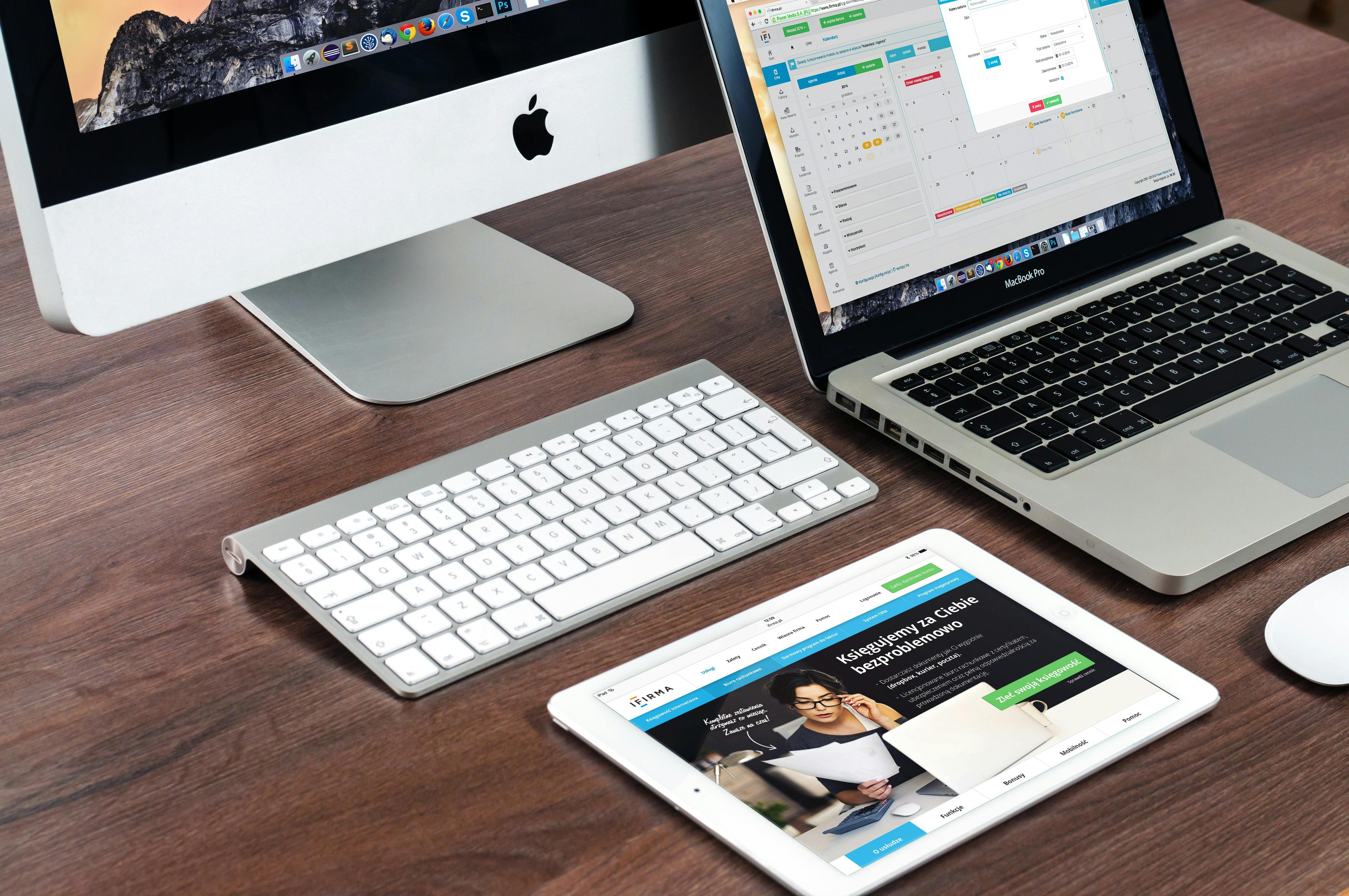
Astro components are at the heart of building efficient and maintainable websites with Astro.js. In this tutorial, we’ll dive deep into creating and using Astro components, covering everything from the basics to advanced techniques.
What are Astro Components?
Astro components are the building blocks of your Astro project. They are files with a .astro
extension that contain reusable pieces of HTML, CSS, and JavaScript.
Basic Structure of an Astro Component
Here’s a simple example of an Astro component:
---
// Component Script (optional)
const greeting = "Hello, Astro!";
---
<!-- Component Template -->
<h1>{greeting}</h1>
<style>
/* Component Styles */
h1 {
color: blue;
}
</style>
Creating Your First Astro Component
Let’s create a simple “Button” component:
- Create a new file called
Button.astro
in yoursrc/components/
directory. - Add the following code:
---
const { text, href } = Astro.props;
---
<a href={href} class="button">
{text}
</a>
<style>
.button {
display: inline-block;
padding: 10px 20px;
background-color: #4CAF50;
color: white;
text-decoration: none;
border-radius: 5px;
transition: background-color 0.3s ease;
}
.button:hover {
background-color: #45a049;
}
</style>
- Now you can use this component in any of your Astro pages like this:
---
import Button from '../components/Button.astro';
---
<Button text="Click me!" href="/about" />
Advanced Techniques
Slots
Slots allow you to pass content to your component. Here’s an example:
---
// Card.astro
---
<div class="card">
<slot name="header" />
<slot />
<slot name="footer" />
</div>
<style>
.card {
border: 1px solid #ccc;
border-radius: 4px;
padding: 20px;
}
</style>
You can use this component like this:
---
import Card from '../components/Card.astro';
---
<Card>
<h2 slot="header">Card Title</h2>
<p>This is the main content of the card.</p>
<small slot="footer">Card footer</small>
</Card>
Dynamic Components
Astro allows you to dynamically select which component to render:
---
import MyComponent from './MyComponent.astro';
const components = {
'my-component': MyComponent,
};
const selectedComponent = 'my-component';
---
<div>
{selectedComponent && components[selectedComponent] && (
<Fragment set:html={components[selectedComponent]} />
)}
</div>
Best Practices
- Keep components small and focused: Each component should have a single responsibility.
- Use props for flexibility: Make your components configurable with props.
- Leverage Astro’s partial hydration: Use client directives like
client:load
orclient:visible
wisely to minimize JavaScript sent to the browser. - Optimize for performance: Use Astro’s built-in asset optimization features for images and other assets.
Conclusion
Astro components are powerful tools for building fast, efficient websites. By mastering their creation and use, you’ll be well on your way to becoming an Astro expert. Happy coding!