Getting Started with Astro.js: A Beginner's Guide
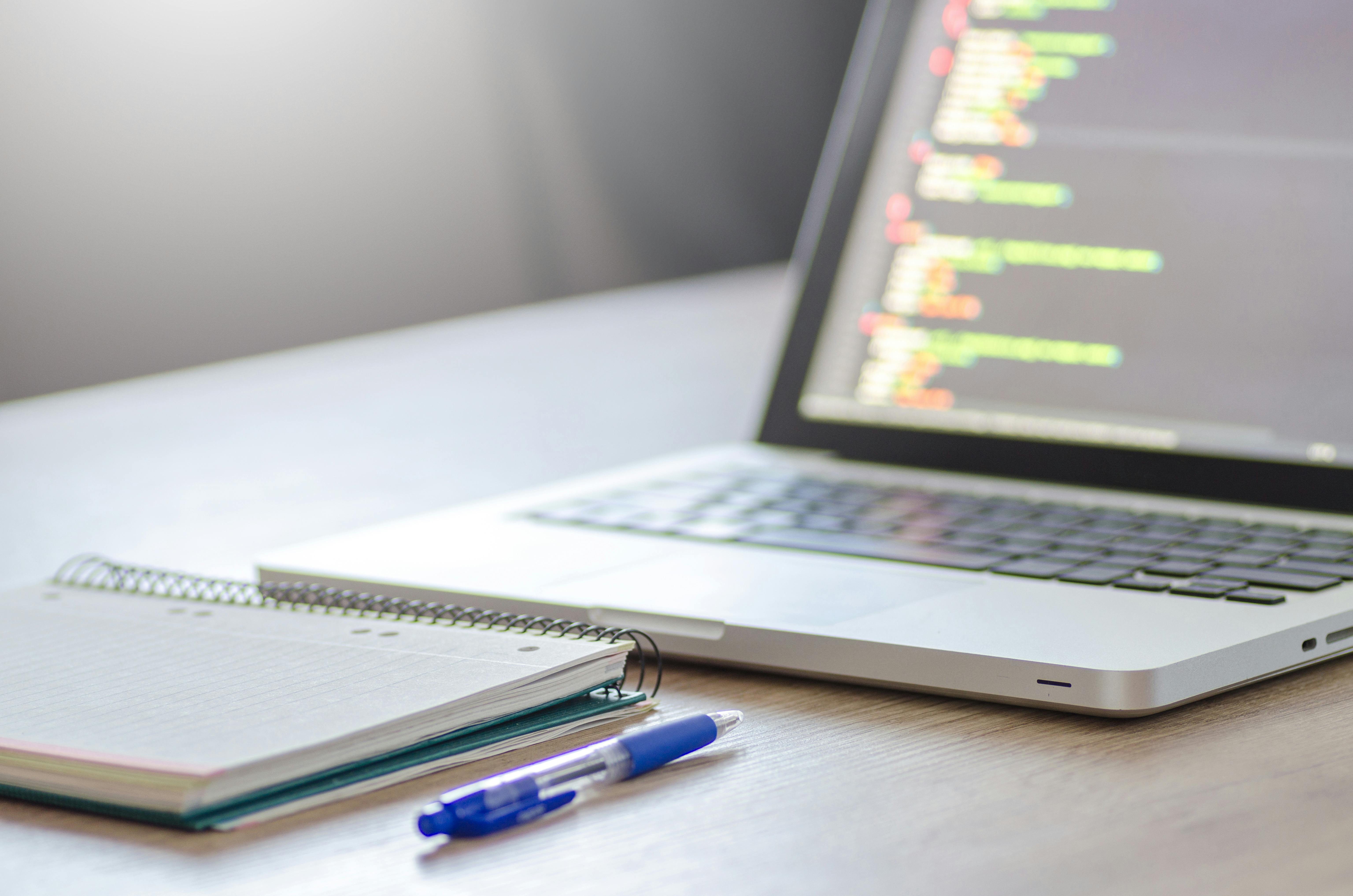
Astro.js is a modern static site generator that allows you to build faster websites with less client-side JavaScript. In this guide, we’ll walk you through setting up your first Astro project and explain some of its core concepts.
Introduction
Astro.js has been gaining popularity among developers for its unique approach to building websites. It combines the best of static site generation with the flexibility of modern JavaScript frameworks. Some key benefits of using Astro include:
- Faster page loads due to minimal JavaScript
- Support for multiple frontend frameworks in a single project
- Easy integration of dynamic content
- Built-in performance optimizations
Whether you’re a seasoned developer or just starting your web development journey, Astro offers a refreshing and efficient way to build websites.
Installation
Before we begin, make sure you have Node.js (version 14.18.0 or higher) and npm installed on your machine. To create a new Astro project, open your terminal and run the following command:
npm create astro@latest
This command will initiate the Astro setup wizard. Follow the prompts to configure your project:
- Enter a name for your project directory.
- Choose whether to create a new directory or use the current one.
- Select a starter template (you can choose the “Empty” option for this tutorial).
- Decide if you want to initialize a git repository.
- Choose whether to install dependencies now or later.
Once the setup is complete, navigate to your project directory and start the development server:
cd your-project-name
npm run dev
Your Astro site is now running locally at http://localhost:3000
!
Project Structure
Let’s take a look at the basic structure of an Astro project:
your-project-name/
├── public/
├── src/
│ ├── components/
│ ├── layouts/
│ └── pages/
├── astro.config.mjs
├── package.json
└── tsconfig.json (if using TypeScript)
public/
: This directory is for static assets that don’t require processing, like images or fonts.src/
: The main source directory for your project.components/
: Reusable Astro components.layouts/
: Layout components for structuring your pages.pages/
: Contains your site’s pages. Each file here becomes a route in your site.
astro.config.mjs
: The configuration file for your Astro project.package.json
: Manages project dependencies and scripts.
Creating Your First Page
Astro uses .astro
files, which are similar to HTML but with added superpowers. Let’s create a simple “Hello World” page:
- Create a new file
src/pages/index.astro
- Add the following content:
---
// Component Script (optional)
const pageTitle = "Welcome to Astro";
---
<html lang="en">
<head>
<meta charset="utf-8" />
<link rel="icon" type="image/svg+xml" href="/favicon.svg" />
<meta name="viewport" content="width=device-width" />
<meta name="generator" content={Astro.generator} />
<title>{pageTitle}</title>
</head>
<body>
<h1>{pageTitle}</h1>
<p>Hello, World!</p>
</body>
</html>
This creates a basic HTML page with a dynamic title. The area between the ---
fences is the component script, where you can write JavaScript that runs at build time.
Adding Styles
Astro provides several ways to add styles to your components. Let’s add some basic styling to our page:
- You can add scoped styles directly in your
.astro
file:
---
const pageTitle = "Welcome to Astro";
---
<html lang="en">
<!-- ... head content ... -->
<body>
<h1>{pageTitle}</h1>
<p>Hello, World!</p>
</body>
</html>
<style>
body {
font-family: Arial, sans-serif;
max-width: 800px;
margin: 0 auto;
padding: 20px;
}
h1 {
color: navy;
}
</style>
- For global styles, you can create a
src/styles/global.css
file and import it in your layout or pages:
/* src/styles/global.css */
body {
background-color: #f0f0f0;
}
Then, in your Astro component:
---
import '../styles/global.css';
// ... rest of the component
---
Using Markdown in Astro
Astro has excellent support for Markdown, making it easy to create content-heavy sites. Let’s add a simple blog post:
- Create a new file
src/pages/first-post.md
- Add the following content:
---
title: My First Blog Post
publishDate: 2024-08-08
description: This is my first blog post using Astro!
---
# Welcome to my Blog
This is my first blog post using Astro. I'm excited to share my thoughts and experiences with you!
## What I've Learned
Astro makes it incredibly easy to create static websites. Here are a few things I've learned:
1. Astro components are powerful and flexible
2. Markdown support is built-in and easy to use
3. The build process is fast and efficient
Stay tuned for more posts as I continue my Astro journey!
Astro will automatically create a new route for this markdown file and render it as HTML.
Building and Deploying
When you’re ready to deploy your site, you can build it using the following command:
npm run build
This will generate a static version of your site in the dist/
directory. You can then deploy this directory to any static hosting platform like Netlify, Vercel, or GitHub Pages.
For example, to deploy to Netlify:
- Push your project to a GitHub repository
- Sign up for a Netlify account and connect it to your GitHub
- Create a new site from your repository
- Set the build command to
astro build
and the publish directory todist
Netlify will automatically build and deploy your site whenever you push changes to your repository.
Next Steps
Congratulations! You’ve created your first Astro site. Here are some next steps to continue your Astro journey:
- Explore Astro’s official documentation for in-depth guides and API references
- Try integrating other frameworks like React or Vue components into your Astro site
- Experiment with Astro’s advanced features like API routes and server-side rendering
Conclusion
In this guide, we’ve covered the basics of getting started with Astro.js. We’ve learned how to set up a new project, create pages, add styles, work with Markdown, and prepare for deployment. Astro’s intuitive design and powerful features make it an excellent choice for building modern, performant websites.
As you continue to explore Astro, you’ll discover even more ways to optimize your development workflow and create amazing web experiences. Happy coding with Astro!